Hi there, welcome to the fourth part of Building API with Rails series. In this part we are going to discuss how we can secure our API.
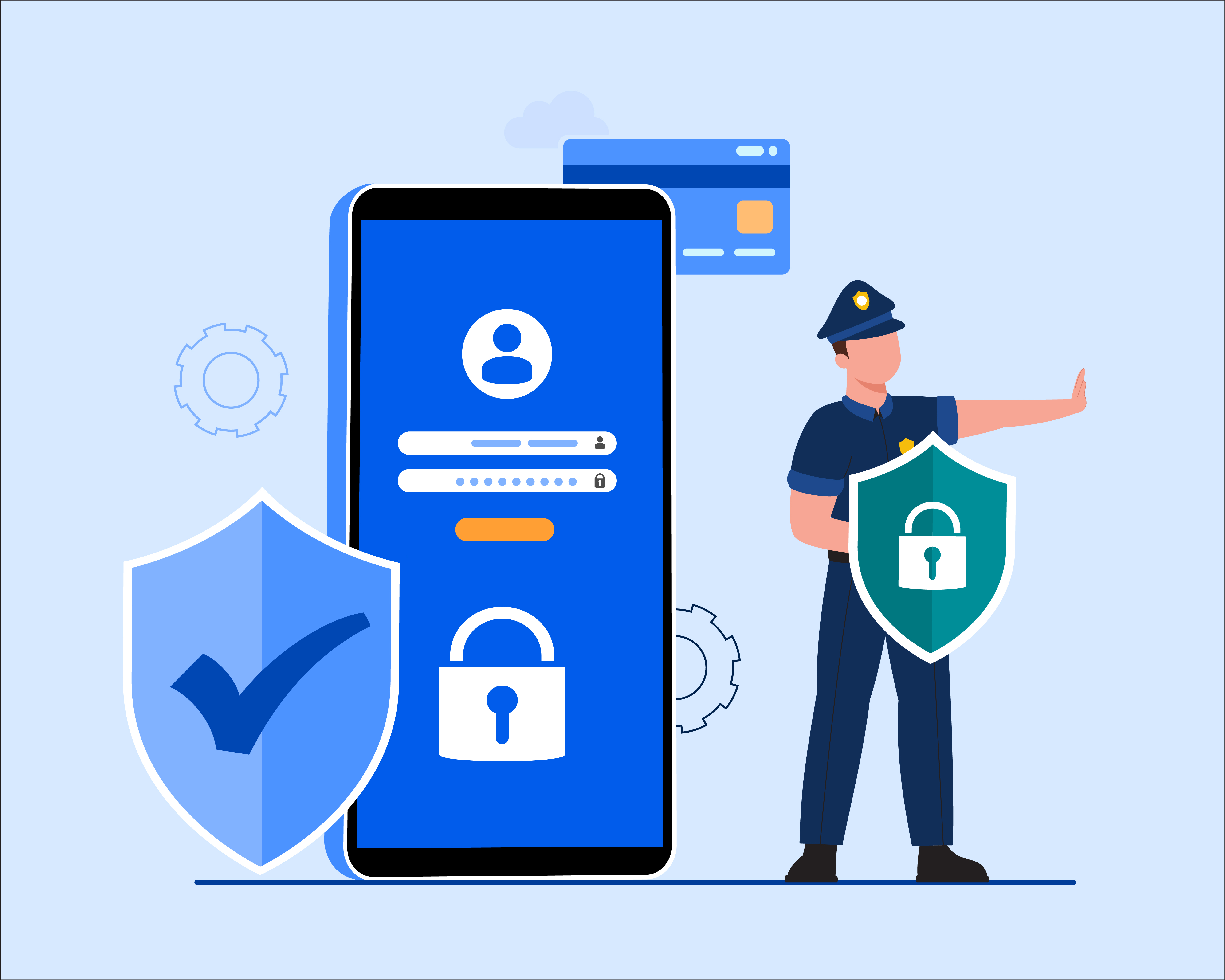
Business vector created by jcomp - www.freepik.com
Normally when it comes to securing API or web application its done through the process of authentication and authoriztion..
There are a mainly two ways for doing the authentication part which is Session based Authentication and Token Based Authentication and one of the best and renown one in Rails for session based authentication is Devise.
For token based authentication we can also ues Devise Token Auth. Simillary, other ones are Knock, Doorkeeper as Oauth provider and so on. I will be using JWT for the token based authentication for this application.
Thanks for reading till the end we have come to the end of part 4 of the Building API with Rails series. In the next part, we will continue the process of securing our API by using JWT.
The code base is available here. 
Please feel free to give your feedback on the comment section below or ping me at or . Have a great time 